Character Special Files (c)
represents hardware devices that reads or writes a serial stream of data bytes. sound cards , terminal devices(tty)
Block speical files (b)
represents hardware devices that raeds or wirtes data in fixed size blocks. HDD, SSD, cdrom
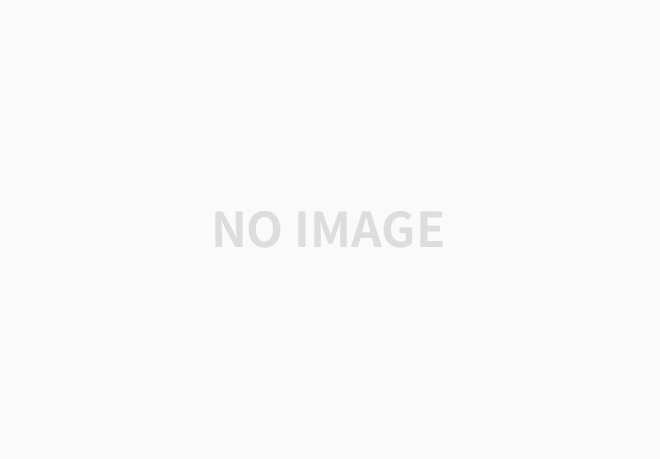
colored boxes are device drvierers , work as an interface so that OS can communciate with devices more formally.
regular file is container , inode block points to its data blocks.
device file is conenction , inode block points to function inside kernel called device driver.
linux identify devices using a 16 bit number divided into two parts
- Major nubmer (8bits) = identifies the driver program
- Minor number (8bits) = used by driver program to identify the instance
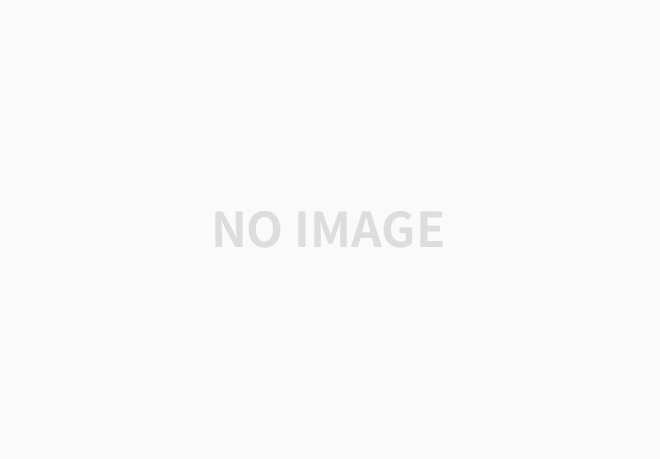
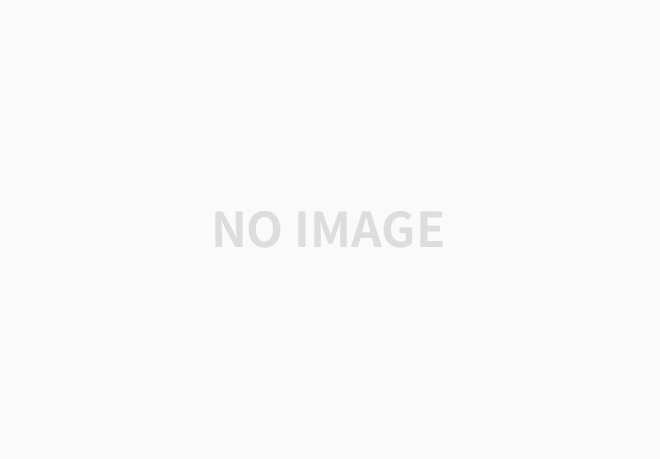
we can write something into other terminal by redirection.
#include <stdio.h>
#include <unistd.h>
#include <fcntl.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]){
if (argc != 2 ){
fprintf(stderr,"usage: ./a.out ttyname\n");
exit(1);
}
int fd = open(argv[1], 1);
if (fd == -1){
perror("open() failed");
exit(1);
}
char buf[512]; /* loop until EOF on input */
while(fgets(buf, 512, stdin) != NULL )
if (write(fd, buf, strlen(buf)) == -1 )
break;
close(fd);
return 0;
}
we open terminal file (character special file) with write mode.
and get input from keyboard and write it back to given fd (terminal file).
Modes of terminal driver
Canonical Mode = input is made available line by line, and the line goest to the process only after the user presses the enter key (buffered inside the tty driver program)
Non Canonical Mode = input is made available immediately as a key is pressed, without the need to press the Enter key (no buffering is done by the driver program)
Attribute of Terminal Driver
Input Processing
Processing performed by tty driver on the characters received via key board, sending them to the prcoess. icrnl
Output Processing
Processing performed by tty driver on the characters received from process, before sending them to the display unit. onclr
Control Processing
How characters are represented? cs8
Local Processing
What the driver do while the characters are inside the driver? icanon, echo
Stty
change and print terminal line settings
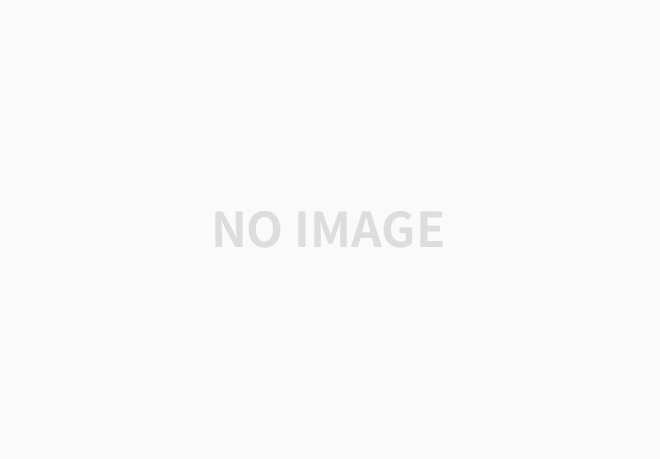
there are lots of flags(options for input and output) that we can manage.
tcgetattr , tcsetattr
#include <stdio.h>
#include <termios.h>
#include <stdlib.h>
#include <string.h>
int main(int argc, char *argv[]){
char passwd[50];
printf("Password:");
//get attributes
struct termios info;
tcgetattr(0, &info);
//save a copy of original attributes
struct termios save = info;
//make the echo bit off and set attributes
info.c_lflag &= ~ECHO;
tcsetattr(0, TCSANOW, &info);
//get password from user
fgets(passwd, 50, stdin);
char *q;
q = strchr(passwd, '\n');
*q = '\0';
//now set the attributes back to original
tcsetattr(0, TCSANOW, &save);
//compare password and print appropriate message
int rv = strcmp(passwd, "pucit");
if (rv == 0)
printf("\nThe password is correct\n");
else
printf("\nThe password is incorrect\n");
return 0;
}
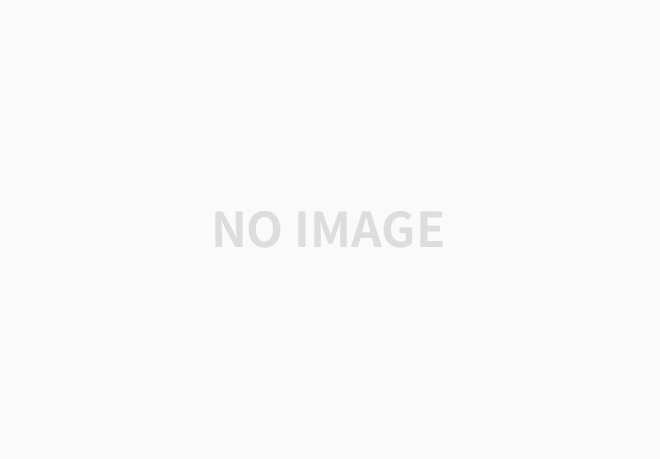
by making the echo bit off , we can hide our password because terminal won't echo what we wrote in keyboard.
tcgetattr's first argument is keyboard because file descriptor zero is standard input.
to open disk file = fcntl system call
to open device file = ioctl system call
'Operating System > System Programming(Arif Butt)' 카테고리의 다른 글
Lec18) Process Management-II (0) | 2021.07.10 |
---|---|
Lec17) Process Management-I (0) | 2021.07.09 |
Lec15) Design and Code Of UNIX who Utility , Buffering (0) | 2021.07.06 |
Lec14) Design and Code of UNIX ls Utility (0) | 2021.07.05 |
Lec13) UNIX File Management (0) | 2021.06.29 |